Introduction
In the world of trading, effective money management is crucial for long-term success. Regardless of the trading strategy employed, poor money management can quickly lead to significant losses and even account devastation. Therefore, developing and implementing robust money management systems is essential for traders seeking sustainable profitability.
In this comprehensive guide, we delve into the realm of money management systems within the context of MQL5, the programming language for the MetaTrader 5 platform. We'll explore various techniques and strategies to manage risk and optimize position sizing, providing both theoretical explanations and practical implementations using MQL5 code examples.
- Disclaimer::
- The material provided here is for educational purposes only and should not be considered financial advice or a recommendation to engage in any trading activity. Trading in financial markets involves risk, including the risk of losing invested capital. Before making any investment decision, you should carefully consider your investment objectives, experience level, and risk appetite. The author shall not be liable for any losses or damages arising from the use of the information provided here.
Understanding Money Management Principles
Risk management strategies are fundamental principles employed by traders to minimize potential losses and protect their capital. These strategies aim to control the amount of capital exposed to risk in any given trade or series of trades. Effective risk management is crucial for long-term success in trading and involves various techniques and practices.
Before diving into the specifics, let's briefly review what money management entails in the context of trading. Money management refers to the process of allocating capital, determining position sizes, setting stop-loss levels, and managing risk to preserve capital and maximize returns. The significance of money management cannot be overstated. While trading strategies are essential for identifying potential opportunities in the market, effective money management ensures that these opportunities are capitalized upon while minimizing the inherent risks associated with trading.
In the subsequent sections, we will explore various money management systems, elucidate their principles, and demonstrate their implementation in MQL5 through code examples. By the end of this guide, you'll have a comprehensive understanding of how to integrate effective money management strategies into your trading algorithms using MQL5.
Risk management
Setting a maximum risk per trade: Diversifying a trading portfolio across different assets or trading strategies can help spread risk and reduce the impact of adverse market movements on overall performance.
Diversification: Diversifying a trading portfolio across different asset classes, markets, or trading strategies can help spread risk and reduce the impact of adverse market movements on overall performance.
Using stop-loss orders: Implementing stop-loss orders allows traders to define exit points for their trades, limiting losses if the market moves against them beyond a certain point.
Monitoring leverage: Controlling the amount of leverage used in trading is essential for managing risk. While leverage can amplify potential profits, it also increases the risk of significant losses, so it's important to use it judiciously.
Position Sizing Techniques
Position-sizing techniques are crucial for managing risk and optimizing returns in trading. They determine the optimal size of each position based on various factors, such as account size, risk tolerance, and market conditions. Here are two common position-sizing implementation techniques:
Fixed Lot Size: This technique involves trading a fixed number of lots or contracts for each trade, regardless of account size or market conditions. It's simple to implement but may not account for changes in volatility or account equity.
- Code:
//+------------------------------------------------------------------+
//| FixLotSizing.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
/*
This MQL5 script demonstrates the basic implementation of a fixed lot size trading strategy. It includes dummy functions for position adjustment,
opening buy and sell positions, as well as validating stop-loss and take-profit levels. Please note that this is just a simplified example for educational purposes,
showcasing how a fixed lot size strategy can be applied. The script generates random trading signals and executes trades based on predefined parameters,
aiming to illustrate the concept of fixed lot size trading.
Author: Dragan Drenjanin
Date: February 09, 2024
*/
//+------------------------------------------------------------------+
// Enumeration for different trading signals
enum TradingSignal {
SIGNAL_NONE, // No signal
SIGNAL_BUY, // Buy signal
SIGNAL_SELL // Sell signal
};
// Input variable for fixed lot size
input double FixedLotValue = 0.01; // Default value 0.01
//+------------------------------------------------------------------+
// Function to adjust position size based on symbol properties
double AdjustPositionSize(double size) {
string symbol = Symbol(); // Get the current symbol
double Smallest_Lot = SymbolInfoDouble(symbol, SYMBOL_VOLUME_MIN);
double LotStep = SymbolInfoDouble(symbol, SYMBOL_VOLUME_STEP);
double adjustedSize = MathRound(size / LotStep) * LotStep;
if (adjustedSize < Smallest_Lot) {
Print("Adjusted position size (", DoubleToString(adjustedSize), ") is lower than the minimum possible (", DoubleToString(Smallest_Lot), "). Using the minimum lot size...");
return Smallest_Lot; }
else {
return adjustedSize; } }
//+------------------------------------------------------------------+
// Function to open a buy position
void OpenBuyPosition(double volume, double takeProfit, double stopLoss) {
// Check if there is already an open position
if (IsPositionOpened()) {
Print("There is already an open position. Skipping buy order placement.");
return; }
MqlTradeRequest request = { };
MqlTradeResult result = { };
// Set parameters for the buy request
request.action = TRADE_ACTION_DEAL;
request.symbol = Symbol();
request.volume = volume;
request.type = ORDER_TYPE_BUY;
request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK);
request.deviation = 5;
request.magic = 123;
request.tp = takeProfit;
request.sl = stopLoss;
// Send the buy request
if (!OrderSend(request, result))
PrintFormat("Error placing buy order! Error code: %d", GetLastError());
else
PrintFormat("Buy order placed successfully! Order ticket: %I64u", result.order); }
//+------------------------------------------------------------------+
// Function to open a sell position
void OpenSellPosition(double volume, double takeProfit, double stopLoss) {
// Check if there is already an open position
if (IsPositionOpened()) {
Print("There is already an open position. Skipping sell order placement.");
return; }
MqlTradeRequest request = { };
MqlTradeResult result = { };
// Set parameters for the sell request
request.action = TRADE_ACTION_DEAL;
request.symbol = Symbol();
request.volume = volume;
request.type = ORDER_TYPE_SELL;
request.price = SymbolInfoDouble(Symbol(), SYMBOL_BID);
request.deviation = 5;
request.magic = 123;
request.tp = takeProfit;
request.sl = stopLoss;
// Send the sell request
if (!OrderSend(request, result))
PrintFormat("Error placing sell order! Error code: %d", GetLastError());
else
PrintFormat("Sell order placed successfully! Order ticket: %I64u", result.order); }
//+------------------------------------------------------------------+
void OnTick() {
// Initialize variables for trading signal
int signal = GetTradingSignal();
// If it's a buy signal
if (signal == SIGNAL_BUY) {
double ask = SymbolInfoDouble(Symbol(), SYMBOL_ASK);
double stopLoss = NormalizeDouble(ask - 100 * Point(), _Digits); // Set stop loss 100 pips below current ask
double takeProfit = NormalizeDouble(ask + 200 * Point(), _Digits); // Set take profit 200 pips above current ask
// Check if stop loss and take profit are within valid range
if (!IsStopLossTakeProfitValid(stopLoss, takeProfit)) {
Print("Invalid stop loss or take profit. Skipping buy order placement.");
return; }
double volume = AdjustPositionSize(FixedLotValue);
OpenBuyPosition(volume, takeProfit, stopLoss); }
// If it's a sell signal
else if (signal == SIGNAL_SELL) {
double bid = SymbolInfoDouble(Symbol(), SYMBOL_BID);
double stopLoss = NormalizeDouble(bid + 100 * Point(), _Digits); // Set stop loss 100 pips above current bid
double takeProfit = NormalizeDouble(bid - 200 * Point(), _Digits); // Set take profit 200 pips below current bid
// Check if stop loss and take profit are within valid range
if (!IsStopLossTakeProfitValid(stopLoss, takeProfit)) {
Print("Invalid stop loss or take profit. Skipping sell order placement.");
return; }
double volume = AdjustPositionSize(FixedLotValue);
OpenSellPosition(volume, takeProfit, stopLoss); } }
//+------------------------------------------------------------------+
// Function to check if stop loss and take profit are valid
bool IsStopLossTakeProfitValid(double stopLoss, double takeProfit) {
// Check if stop loss is less than take profit
if (stopLoss >= takeProfit) {
Print("Stop loss must be less than take profit.");
return false; }
// Add any additional checks for validity here
// For example, you can check if stop loss and take profit are within a certain range
return true; }
//+------------------------------------------------------------------+
// Function to get a random trading signal
int GetTradingSignal() {
int randomValue = MathRand() % 3;
if (randomValue == 0) {
return SIGNAL_BUY; }
else if (randomValue == 1) {
return SIGNAL_SELL; }
else {
return SIGNAL_NONE; } }
//+------------------------------------------------------------------+
// Function to check if there is already an open position
bool IsPositionOpened() {
int totalPositions = PositionsTotal();
for (int i = 0; i < totalPositions; i++) {
ulong ticket = PositionGetTicket(i);
if (ticket != 0) {
return true; // Return true if any non-zero ticket is found
} }
return false; // Return false if no non-zero tickets are found
}
//+------------------------------------------------------------------+
Percentage Risk: With this technique, the position size is determined based on a percentage of the trader's account equity or capital. For example, risking 1% of the account equity on each trade ensures consistent risk management, but position sizes may vary depending on account size and market volatility.
- Code:
//+------------------------------------------------------------------+
//| Percentage Risk.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
/* Description
This code is a simple trading algorithm implemented in MQL5 for the MetaTrader 5 platform.
It defines a maximum risk per trade, stop loss and take profit distances, and calculates position sizes based on risk management principles.
The algorithm generates trading signals randomly (buy, sell, or none) and opens positions accordingly.
It includes functions to open buy and sell positions, calculate position sizes, check for existing positions, and generate random trading signals.
Each function is documented with comments explaining its purpose and usage.
The algorithm is designed to be used as a template or educational example for traders and developers looking to implement basic trading strategies in the MQL5 language.
It emphasizes the importance of risk management and position sizing in trading systems.
Author: Dragan Drenjanin
Date: February 09, 2024
*/
//+------------------------------------------------------------------+
// Enumeration for different trading signals
enum TradingSignal {
SIGNAL_NONE, // No signal
SIGNAL_BUY, // Buy signal
SIGNAL_SELL // Sell signal
};
//+------------------------------------------------------------------+
// Define maximum risk per trade, decrease factor, stop loss, and take profit distances
input double MaxRiskPerTrade = 2.0; // Max risk per trade in percentage (starting range: 0.01.....)
input double DecreaseFactor = 6.7; // Decrease factor for position sizing (starting range: 0.01.....)
input double StopLossDistance = 10.0; // Stop loss distance in pips
input double TakeProfitDistance = 50.0; // Take profit distance in pips
//--- Value for ORDER_MAGIC
input long order_magic=55555;
//+------------------------------------------------------------------+
// Function to calculate position size
double CalculatePositionSize(string symbol, double mMaximumRisk, double mDecreaseFactor) {
double price = 0.0;
double margin = 0.0;
//--- Select lot size
if(!SymbolInfoDouble(_Symbol, SYMBOL_ASK, price))
return(0.0);
if(!OrderCalcMargin(ORDER_TYPE_BUY, _Symbol, 1.0, price, margin))
return(0.0);
if(margin <= 0.0)
return(0.0);
double lot = NormalizeDouble(AccountInfoDouble(ACCOUNT_MARGIN_FREE) * mMaximumRisk / 100000.0, _Digits);
//--- Calculate number of consecutive losing trades
if(mDecreaseFactor > 0) {
//--- Select history for access
HistorySelect(0, TimeCurrent());
//--- Initialize variables
int orders = HistoryDealsTotal(); // Total number of history deals
int losses = 0; // Number of consecutive losing trades
//--- Loop through trade history
for(int i = orders - 1; i >= 0; i--) {
ulong ticket = HistoryDealGetTicket(i);
if(ticket == 0) {
Print("HistoryDealGetTicket failed, no trade history");
break; }
//--- Check symbol
if(HistoryDealGetString(ticket, DEAL_SYMBOL) != _Symbol)
continue;
//--- Check Expert Magic number
if(HistoryDealGetInteger(ticket, DEAL_MAGIC) != order_magic)
continue;
//--- Check profit
double profit = HistoryDealGetDouble(ticket, DEAL_PROFIT);
if(profit > 0.0)
break;
if(profit < 0.0)
losses++; }
//--- Decrease lot size based on consecutive losses
if(losses > 1)
lot = NormalizeDouble(lot - lot * losses / DecreaseFactor, 1); }
//--- Normalize and check limits
double stepvol = SymbolInfoDouble(_Symbol, SYMBOL_VOLUME_STEP);
lot = stepvol * NormalizeDouble(lot / stepvol, 0);
double minvol = SymbolInfoDouble(_Symbol, SYMBOL_VOLUME_MIN);
if(lot < minvol)
lot = minvol;
double maxvol = SymbolInfoDouble(_Symbol, SYMBOL_VOLUME_MAX);
if(lot > maxvol)
lot = maxvol;
//--- Return trading volume
return(lot); }
//+------------------------------------------------------------------+
// Function to open a buy position
void OpenBuyPosition(double volume, double takeProfit, double stopLoss) {
// Check if there is already an open position
if (IsPositionOpened()) {
Print("There is already an open position. Skipping buy order placement.");
return; }
MqlTradeRequest request = { };
MqlTradeResult result = { };
// Set parameters for the buy request
request.action = TRADE_ACTION_DEAL;
request.symbol = Symbol();
request.volume = volume;
request.type = ORDER_TYPE_BUY;
request.price = SymbolInfoDouble(Symbol(), SYMBOL_ASK);
request.deviation = 5;
request.magic = order_magic;
request.tp = SymbolInfoDouble(Symbol(), SYMBOL_ASK) + takeProfit * Point();
request.sl = SymbolInfoDouble(Symbol(), SYMBOL_ASK) - stopLoss * Point();
// Send the buy request
if (!OrderSend(request, result))
PrintFormat("Error placing buy order! Error code: %d", GetLastError());
else
PrintFormat("Buy order placed successfully! Order ticket: %I64u", result.order); }
//+------------------------------------------------------------------+
// Function to open a sell position
void OpenSellPosition(double volume, double takeProfit, double stopLoss) {
// Check if there is already an open position
if (IsPositionOpened()) {
Print("There is already an open position. Skipping sell order placement.");
return; }
MqlTradeRequest request = { };
MqlTradeResult result = { };
// Set parameters for the sell request
request.action = TRADE_ACTION_DEAL;
request.symbol = Symbol();
request.volume = volume;
request.type = ORDER_TYPE_SELL;
request.price = SymbolInfoDouble(Symbol(), SYMBOL_BID);
request.deviation = 5;
request.magic = order_magic;
request.tp = SymbolInfoDouble(Symbol(), SYMBOL_BID) - takeProfit * Point();
request.sl = SymbolInfoDouble(Symbol(), SYMBOL_BID) + stopLoss * Point();
// Send the sell request
if (!OrderSend(request, result))
PrintFormat("Error placing sell order! Error code: %d", GetLastError());
else
PrintFormat("Sell order placed successfully! Order ticket: %I64u", result.order); }
//+------------------------------------------------------------------+
// Usage example
void OnTick() {
// Calculate position size
double positionSize = CalculatePositionSize(_Symbol, MaxRiskPerTrade, DecreaseFactor);
if (positionSize <= 0) {
// Handle the situation where position size is 0 or negative
Print("Position size is invalid. Handle this situation according to your strategy.");
return; // Exit the function
}
// Initialize variable for trading signal
int signal = GetTradingSignal();
// If it's a buy signal
if (signal == SIGNAL_BUY) {
OpenBuyPosition(positionSize, TakeProfitDistance, StopLossDistance); }
// If it's a sell signal
else if (signal == SIGNAL_SELL) {
OpenSellPosition(positionSize, TakeProfitDistance, StopLossDistance); } }
//+------------------------------------------------------------------+
// Function to get a random trading signal
int GetTradingSignal() {
int randomValue = MathRand() % 3;
if (randomValue == 0) {
return SIGNAL_BUY; }
else if (randomValue == 1) {
return SIGNAL_SELL; }
else {
return SIGNAL_NONE; } }
//+------------------------------------------------------------------+
// Function to check if there is already an open position
bool IsPositionOpened() {
int totalPositions = PositionsTotal();
for (int i = 0; i < totalPositions; i++) {
ulong ticket = PositionGetTicket(i);
if (ticket != 0) {
return true; // Return true if any non-zero ticket is found
} }
return false; // Return false if no non-zero tickets are found
}
//+------------------------------------------------------------------+
Let's delve into a practical example of position sizing based on market volatility. Imagine you're a trader aiming to optimize your risk management strategy. One approach involves adjusting your position size according to market conditions, specifically its volatility. We'll explore a function that calculates the position size using the Average True Range (ATR) indicator. This indicator provides insights into the market's volatility, allowing us to tailor our risk exposure accordingly. Let's take a closer look at how this function operates:
- Code:
double CalculateVolatilityPositionSize(double equity, double atrMultiplier, double atrValue)
{
double riskAmount = equity * 0.02; // Risk 2% of equity per trade
double positionSize = riskAmount / (atrMultiplier * atrValue); // Calculate position size based on ATR
return positionSize;
}
Input Parameters and Explanations
- Equity: the current amount of capital or funds in the account.
- ATR Multiplier: The multiplier is used to scale the ATR indicator value.
- ATR Value: The current value of the ATR indicator for a specific period.
- Risk Calculation: Firstly, the function determines the risk amount as 2% of the total capital (equity * 0.02), which is a standard practice for risk management.
- Position Size Calculation: Next, it calculates the position size by dividing the risk amount (riskAmount) by the product of the ATR multiplier and the ATR value (atrMultiplier * atrValue). This step allows scaling the position size based on the current market volatility, aiding in risk management.
- Result: Finally, the function returns the calculated position size, which can be used for further trading actions.
In summary, this function enables the trading algorithm to dynamically adjust the position size based on the current market volatility, which is crucial for effective risk management and performance optimization.
Other Examples Of Money Management Systems
- Fixed Fractional Position Sizing
- Fixed Ratio Position Sizing
- Kelly Criterion
- Optimal "f" Position Sizing
Fixed Fractional Position Sizing
Fixed Fractional Position Sizing is a popular money management technique wherein the size of each trade is determined based on a fixed percentage of the trader's equity or capital. This approach aims to balance risk and reward by allocating a consistent proportion of capital to each trade, regardless of the account size.
The formula for calculating the position size using Fixed Fractional Position Sizing is:
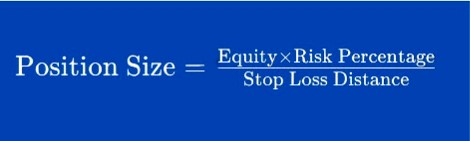
Where:
Equity: The current equity or account balance.
Risk Percentage: The percentage of equity that the trader is willing to risk on a single trade.
Stop Loss Distance: The distance in pips between the entry price and the stop-loss level.
By using fixed fractional position sizing, traders can effectively manage their risk exposure while allowing for the potential growth of their trading capital over time.
Implementation Example in MQL5:
- Code:
//+------------------------------------------------------------------+
//| Fixed Fractional Position Sizing.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
// Define risk percentage and stop loss distance
input double RiskPercentage = 2.0; // Risk 2% of equity per trade
input int StopLossDistance = 100; // Stop loss distance in pips
// Calculate position size based on fixed fractional position sizing
double CalculatePositionSize(double equity, double price, double stopLoss) {
double riskAmount = equity * RiskPercentage / 100.0;
double pipValue = NormalizeDouble(SymbolInfoDouble(Symbol(), SYMBOL_POINT), _Digits);
double positionSize = riskAmount / (stopLoss * pipValue);
return positionSize; }
// Usage example
void OnTick() {
double Ask = SymbolInfoDouble(Symbol(), SYMBOL_ASK);
double equity = AccountInfoDouble(ACCOUNT_EQUITY); // Get current account equity
double stopLoss = Ask - StopLossDistance * Point(); // Calculate stop loss level
stopLoss = NormalizeDouble(stopLoss, _Digits); // Normalize stop loss level
double positionSize = CalculatePositionSize(equity, Ask, StopLossDistance);
// Place trade with calculated position size
// (Implementation of trade execution omitted for brevity)
}
//+------------------------------------------------------------------+
In this example, we define the desired risk percentage and stop-loss distance as inputs. The CalculatePositionSize function then calculates the position size based on the current equity, risk percentage, and stop-loss distance. Finally, in the OnTick function (assuming this code is executed on every tick), we obtain the current equity and calculate the position size using the defined parameters, ready to be used for trade execution.
Fixed Ratio Position Sizing
Fixed Ratio Position Sizing is another money management technique that determines the size of each trade based on a fixed ratio of the trader's equity or capital. Unlike fixed fractional position sizing, which allocates a fixed percentage of equity per trade, fixed ratio position sizing allocates a fixed ratio of equity per trade.
The formula for calculating the position size using fixed ratio position sizing is:

Implementation Example in MQL5
- Code:
//+------------------------------------------------------------------+
//| Fixed Ratio Position Sizing.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
// Define fixed ratio and stop loss distance
input double FixedRatio = 0.02; // Allocate 2% of equity per trade
input int StopLossDistance = 100; // Stop loss distance in pips
// Calculate position size based on fixed ratio position sizing
double CalculatePositionSize(double equity, double price, double stopLoss) {
double positionSize = (equity * FixedRatio) / (stopLoss * Point());
return positionSize; }
// Usage example
void OnTick() {
double Ask = SymbolInfoDouble(Symbol(), SYMBOL_ASK);
double equity = AccountInfoDouble(ACCOUNT_EQUITY); // Get current account equity
double stopLoss = Ask - StopLossDistance * Point(); // Calculate stop loss level
stopLoss = NormalizeDouble(stopLoss, _Digits); // Normalize stop loss level
double positionSize = CalculatePositionSize(equity, Ask, StopLossDistance);
// Place trade with calculated position size
// (Implementation of trade execution omitted for brevity)
In this example, we define the fixed ratio (percentage) of equity to be allocated per trade and the stop-loss distance in pips as inputs. The CalculatePositionSize function then calculates the position size based on the current equity, fixed ratio, and stop loss distance. Similarly to the previous example, in the OnTick function, we obtain the current equity and calculate the position size using the defined parameters, ready to be used for trade execution.
Kelly Criterion
The Kelly criterion is a mathematical formula used to determine the optimal position size for a series of trades, taking into account the probability of success and the potential payoff. Developed by John L. Kelly Jr. in the 1950s, the Kelly Criterion aims to maximize the growth rate of a trader's capital over time while minimizing the risk of ruin.
The formula for calculating the Kelly fraction (the portion of capital to allocate to each trade) is as follows:

Implementation Example in MQL5
- Code:
//+------------------------------------------------------------------+
//| Kelly Criterion.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
// Define odds, probability of success, and probability of failure
input double Odds = 2.0; // Odds received on the bet
input double ProbabilitySuccess = 0.6; // Probability of success
double ProbabilityFailure = 1.0 - ProbabilitySuccess; // Probability of failure
// Calculate Kelly fraction based on Kelly Criterion
double CalculateKellyFraction(double odds, double probabilitySuccess) {
double kellyFraction = (odds * probabilitySuccess - ProbabilityFailure) / odds;
return kellyFraction; }
// Usage example
void OnTick() {
double kellyFraction = CalculateKellyFraction(Odds, ProbabilitySuccess);
// Calculate position size based on Kelly fraction and available equity
double equity = AccountInfoDouble(ACCOUNT_EQUITY); // Get current account equity
double positionSize = equity * kellyFraction;
// Place trade with calculated position size
// (Implementation of trade execution omitted for brevity)
}
//+------------------------------------------------------------------+
Optimal "f" Position Sizing
Optimal "f" Position Sizing is a money management approach that aims to determine the optimal fraction of capital to allocate to each trade, maximizing the growth rate of the trading account while simultaneously minimizing the risk of ruin. Unlike fixed fractional or fixed ratio position sizing, which uses predetermined percentages or ratios, optimal "f" position sizing dynamically adjusts the position size based on the current market conditions and account equity.
The optimal f formula was introduced by Ralph Vince and is derived from the Kelly criterion. It determines the fraction of the account equity to invest in each trade based on the expected return, the probability of success, and the volatility of the trading system.
The formula for calculating the Optimal f fraction is as follows:

Where:
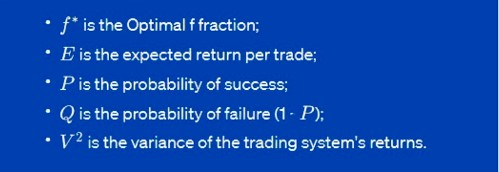
Implementation Example in MQL5
- Code:
//+------------------------------------------------------------------+
//| Optimal f Position Sizing.mq5 |
//| Copyright 2000-2024, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2000-2024, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
// Define expected return, probability of success, and variance
input double ExpectedReturn = 50.0; // Expected return per trade in currency units
input double ProbabilitySuccess = 0.6; // Probability of success
double ProbabilityFailure = 1.0 - ProbabilitySuccess; // Probability of failure
input double Variance = 1000.0; // Variance of the trading system's returns
// Calculate Optimal f fraction based on Optimal f Position Sizing formula
double CalculateOptimalFFraction(double expectedReturn, double probabilitySuccess, double variance) {
double optimalFFraction = (expectedReturn * probabilitySuccess - ProbabilityFailure) / MathPow(variance, 2.0);
return optimalFFraction; }
// Usage example
void OnTick() {
double optimalFFraction = CalculateOptimalFFraction(ExpectedReturn, ProbabilitySuccess, Variance);
// Calculate position size based on Optimal f fraction and available equity
double equity = AccountInfoDouble(ACCOUNT_EQUITY); // Get current account equity
double positionSize = equity * optimalFFraction;
// Place trade with calculated position size
// (Implementation of trade execution omitted for brevity)
}
//+------------------------------------------------------------------+
In this example, we define the expected return per trade, the probability of success, and the variance of the trading system's returns as inputs. The CalculateOptimalFraction function then calculates the optimal f fraction based on these inputs and the predefined probability of failure. In the OnTick function, we obtain the optimal f fraction using the defined parameters and calculate the position size based on the current account equity and the optimal f fraction, ready to be used for trade execution.
Analysis
Equity curve analysis is a critical aspect of evaluating the performance of a trading system over time. It involves studying the historical equity curve, which represents the cumulative profit or loss generated by the trading system as trades are executed. Here are some key aspects of equity curve analysis:
- Visual Inspection: The first step in equity curve analysis is to visually inspect the equity curve. Plotting the equity curve over time allows traders to observe trends, patterns, and characteristics of the trading system's performance.
- Performance Metrics: Various performance metrics can be derived from the equity curve to quantify the performance of the trading system.
These metrics include:
- Total Return: The overall return generated by the trading system over the entire period.
- Maximum Drawdown: The largest peak-to-trough decline in equity experienced by the trading system.
- Sharpe ratio: a measure of risk-adjusted return that considers the volatility of returns.
- Profit Factor: The ratio of total profit to total loss generated by the trading system.
- Win Rate: The percentage of winning trades out of total trades executed.
Stability and Consistency: Equity curve analysis helps assess the stability and consistency of the trading system's performance. A stable equity curve with consistent returns indicates the robustness and reliability of the trading system.
By analyzing the equity curve, traders can identify periods of underperformance or drawdowns and make necessary adjustments to optimize the trading system. This may involve refining trading rules, risk management techniques, or market conditions.
Equity curve analysis is often performed during backtesting (historical simulation) and forward testing (real-time simulation) of the trading system. Backtesting allows traders to evaluate the performance of the system using historical data, while forward testing validates the system's performance in real-market conditions.
This analysis enables traders to identify strengths and weaknesses in their approach and make necessary adjustments to improve performance.
Conclusion
In conclusion, effective money management is essential for successful trading. By implementing robust money management techniques, traders can mitigate risk, optimize returns, and enhance the long-term profitability of their trading strategies. In this guide, we have covered various money management principles, including position sizing techniques such as fixed lot size, percentage risk, volatility-based sizing, and optimal f sizing. Remember that no trading strategy is foolproof, and there will always be risks involved in trading. However, by implementing robust money management practices and continuously monitoring and adjusting your approach, you can enhance your chances of achieving your trading goals and objectives.
Happy trading!
No Comment.